Nimiq Identicons
Visual address verification using Nimiq Identicons
Why Nimiq Identicons?
Protection Against Clipboard Hijacking
In 2019, researchers discovered the first clipper malware on Google Play Store targeting cryptocurrency users. Nimiq Identicons were designed specifically to combat this threat.
The Problem with Crypto Addresses
Traditional cryptocurrency addresses pose two major challenges:
- They are long, complex strings that are difficult to verify
- They are vulnerable to clipboard hijacking attacks
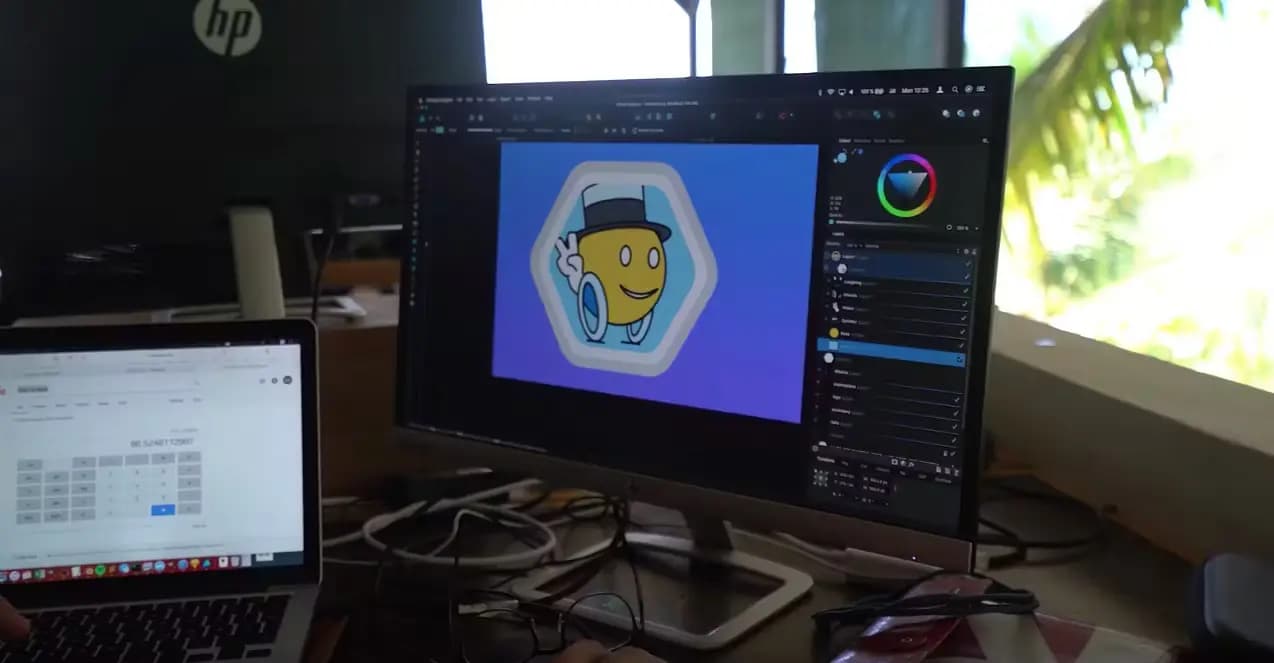
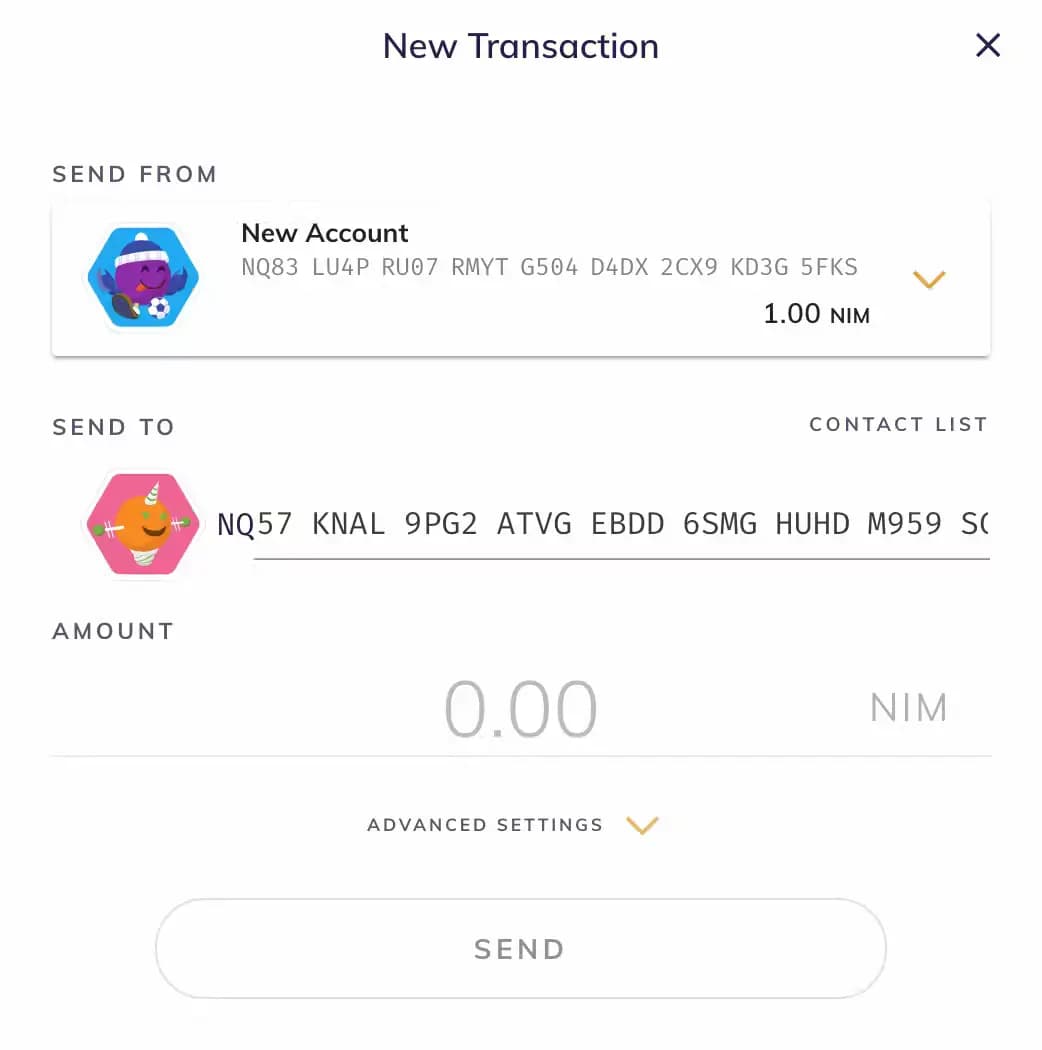
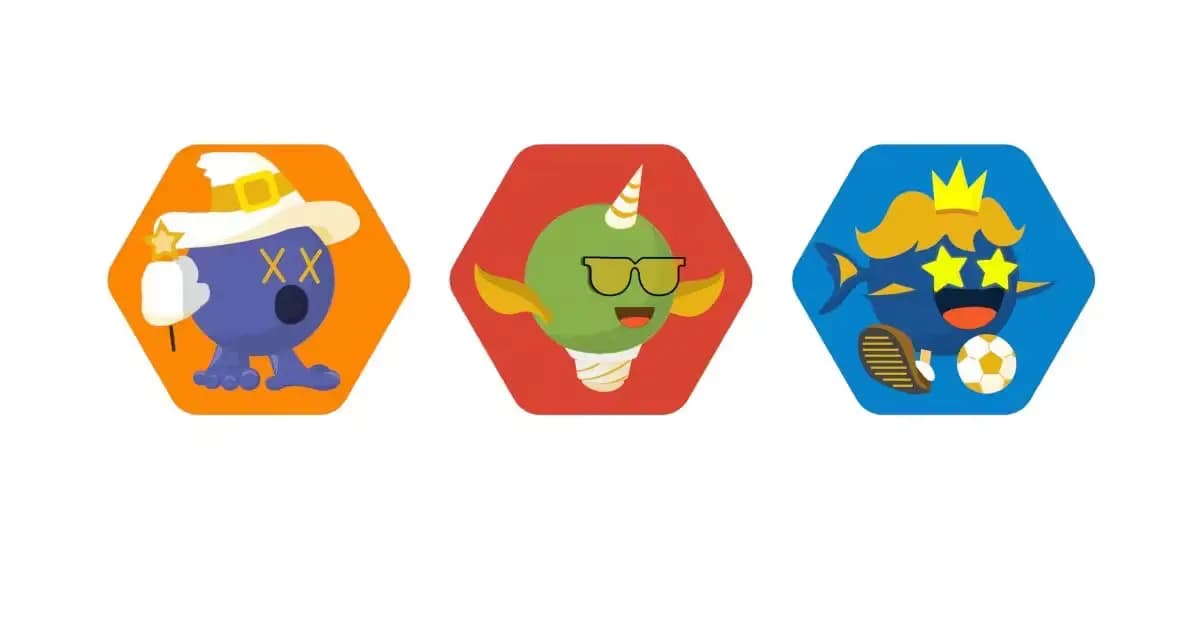
The Solution
"IMHO, too much of the web what we read are textual or numeric information which are not easy to distinguish at a glance when they are jumbled up together. So I think adding visual identifiers will make the user experience much more enjoyable."
— Don Park, creator of the identicon concept
Nimiq Identicons solve these problems by:
- Providing instant visual verification
- Being highly memorable and describable
- Making address tampering immediately obvious
- Supporting natural human pattern recognition
How to Use
Installation
Basic Usage
Create a simple identicon:
Implementation Examples
Advanced Customization
Customize colors and sections:
How It Works
Technical Details
Each Identicon is composed of five distinct elements:
- Bottom shape
- Face features
- Side elements
- Top design
- Color combinations
Components
- 21 different bottoms
- 21 unique faces
- 21 side variations
- 21 top designs
- 8 part colors
- 9 body colors
- 10 background colors
Total Combinations
Over 140 million unique combinations:
10 * 9 * 8 * 21⁴ = 140,026,320
Security Benefits
- Instant Detection: If a clipboard hijacker replaces an address, the Identicon will immediately look different
- Natural Verification: Instead of comparing long strings, users can say "It's the orange unicorn with green eyes"
- Visual Memory: Humans are better at remembering and recognizing visual patterns than text
- Simple Communication: Easily verify addresses over any communication channel
- User-Friendly Security: Protection without complex technical requirements
Real-World Impact
When MetaMask was impersonated on the Google Play Store, the malware could replace crypto addresses in clipboards, affecting millions of users. Nimiq's visual verification system makes such attacks immediately obvious to users.
Migration Guide
SVG Generation
Data URI Generation
Discontinued Features
The following legacy functions are not available in the new implementation:
- makeLetterHash
- wordsByEntropy
- Identicons.render
- Identicons.image
- Identicons.placeholder