Web Client vs RPC Client
Choose the right tool for interacting with the Nimiq blockchain
If you're unsure which client to use for interacting with the Nimiq blockchain, this guide will help you choose the tool that best fits your needs and expertise.
TL;DR
Feature | Web Client | RPC Client |
---|---|---|
Connection | Direct peer connections | Client-server model |
Network Role | Part of blockchain network | Through intermediary node |
Technology | JavaScript/WebAssembly | Language agnostic (HTTP) |
Runtime | Browser and Node.js | Any platform |
Architecture | Lightweight and mobile-friendly | Full-featured server setup |
Getting Started
Web Client
Build client-side applications with direct blockchain interaction. Perfect for web wallets and DApps.
RPC Client
Create backend services and integrations with full node capabilities. Ideal for exchanges and explorers.
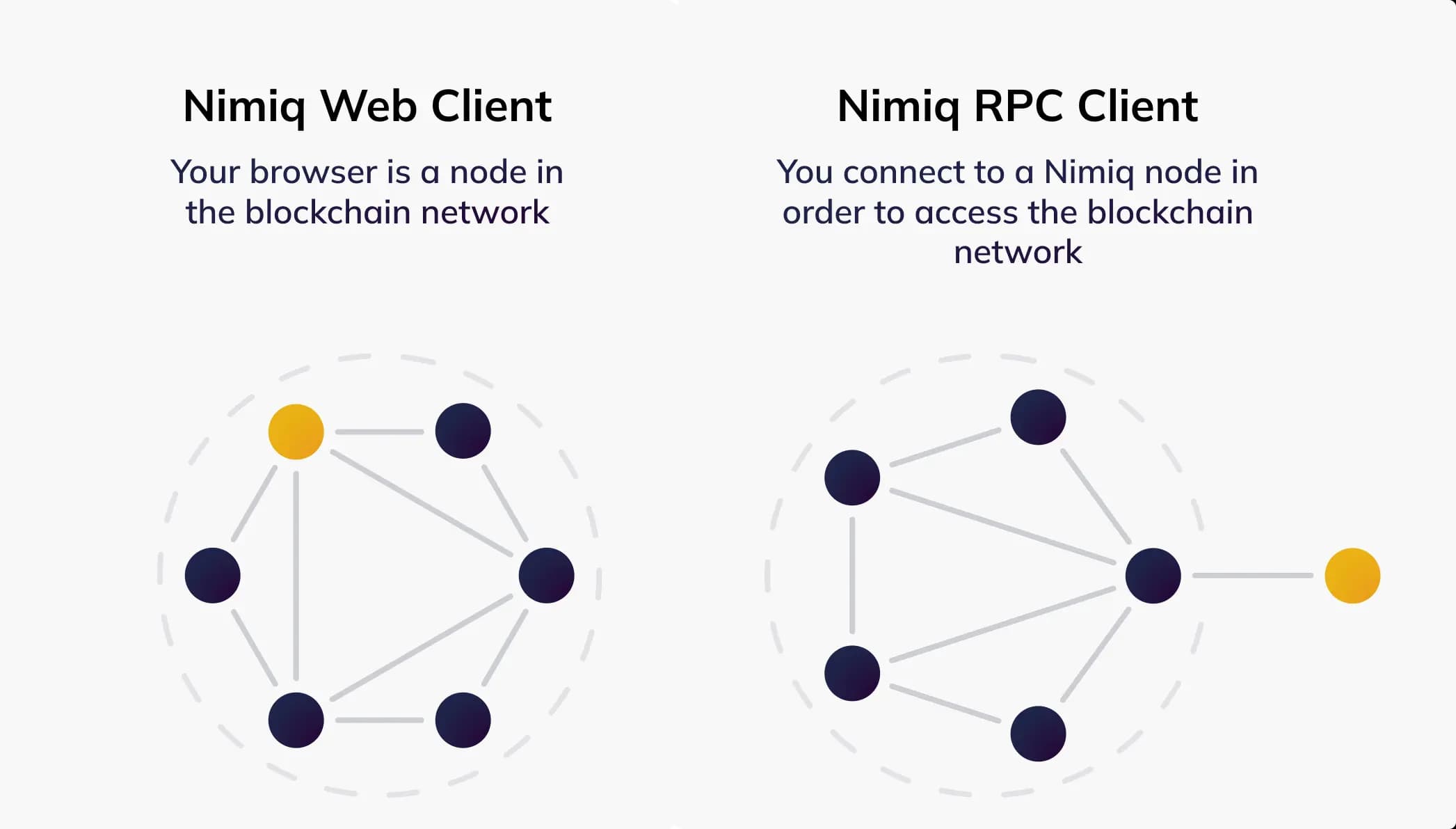
Comparison Overview
Category | Web Client | RPC Client |
---|---|---|
Implementation | JavaScript/WebAssembly | HTTP/JSON-RPC |
Network Model | Direct peer connections | Client-server model |
Environment | Browsers, Node.js, mobile | Any platform |
Best For | Web wallets, DApps | Exchanges, Explorers |
Node Type | Light node | Full/History node |
Setup Time | Minutes (NPM install) | Hours (Node setup) |
Infrastructure | Browser environment | Dedicated server |
Language | JavaScript/TypeScript | Any language |
Target Users | Web developers, end users | System integrators |
Data Access | Recent blockchain data | Complete history |
Detailed Comparison
Web Client
The Web Client is a JavaScript library enabling direct participation in the Nimiq PoS ecosystem:
- Implementation: Rust compiled to WebAssembly using
wasm-pack
- Environment: Browsers, Node.js, mobile devices
- Browser Support: Chrome, Firefox, Edge, Safari
- User Experience: Simple, intuitive interface
- Key Operations:
- Create and manage wallets
- Send transactions
- View transaction history
- Check balances
- Build consensus with peers
Key Features:
- Browser and Node.js compatible
- WebAssembly-powered
- Direct peer connections
- Lightweight consensus
- Simple wallet operations
RPC Client
The RPC provides advanced node access and control:
- Implementation: JSON-RPC over HTTP
- Environment: Any platform with HTTP capabilities
- Access Level: Full node control
- User Experience: Technical, API-focused
- Key Operations:
- Lock/unlock accounts
- Verify transactions
- Manage accounts
- Configure node settings
- Access blockchain state
Use Cases & Operations
Capability | Web Client | RPC Client |
---|---|---|
Wallet Integration | ✓ Web wallets ✓ Simple transactions ✓ Balance checks | ✓ Exchange integration ✓ Complex operations ✓ Account management |
Node Management | ✓ Light consensus ✓ Direct peer connections ✓ Browser-based | ✓ Full node control ✓ Custom configuration ✓ Advanced settings |
Development | ✓ Frontend focused ✓ Quick setup ✓ Mobile friendly | ✓ Backend services ✓ Advanced API access ✓ Multi-language support |
Getting Started
Web Client
Build client-side applications with direct blockchain interaction. Perfect for web wallets and DApps.
RPC Client
Create backend services and integrations with full node capabilities. Ideal for exchanges and explorers.
Learn More
Interested in the technical details? Check out the Nimiq Albatross Protocol Documentation.